Pandas DataFrame DataFrame.where() Function
-
Syntax of
pandas.DataFrame.where()
-
Example Codes:
DataFrame.where()
-
Example Codes:
DataFrame.where()
to Specify a Value -
Example Codes:
DataFrame.where()
to Use Multiple Conditions
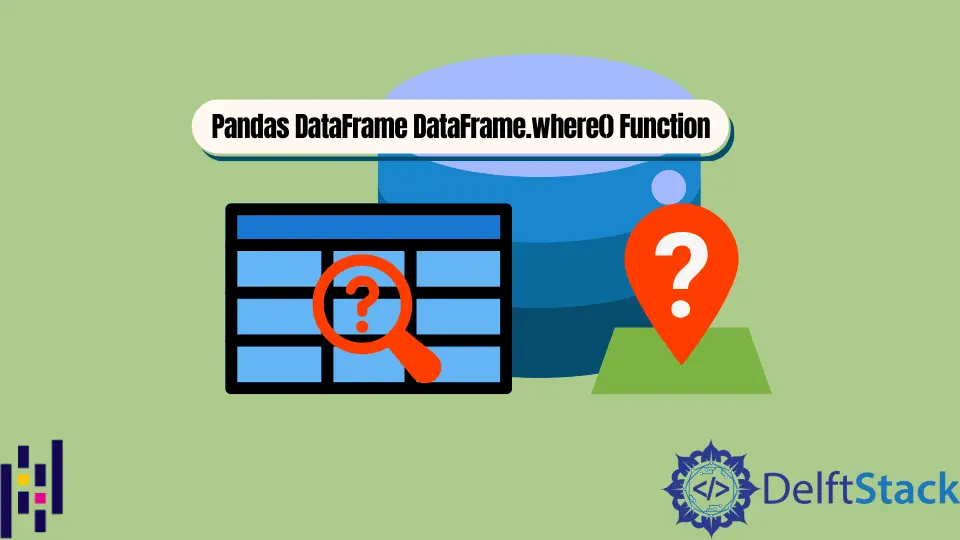
Python Pandas DataFrame.where()
function accepts a condition as a parameter and produces results accordingly. It checks the condition for each value of the DataFrame
and selects the values that accept the condition. The functionality of it is similar to the if-else statement. The value which does not accept the condition is replaced with a NaN
value by default.
Syntax of pandas.DataFrame.where()
DataFrame.where(
cond,
other=NaN,
inplace=False,
axis=None,
level=None,
errors="raise",
try_cast=False,
)
Parameters
This function has several parameters. The default values of all the parameters are mentioned above.
cond |
It is a boolean Series or DataFrame , an array-like structure or, a callable. It represents the condition/conditions to check for each value of the DataFrame . If the condition is True then the original value is not replaced. Otherwise, it is replaced by a NaN value. |
other |
It is a scalar, Series /DataFrame , or a callable. It represents the value that will be placed for the original value if the condition is False . |
inplace |
It is a boolean value. It specifies the operation on data. If True , it makes changes itself. |
axis |
It is an integer value. It specifies the working axis either rows or columns. |
level |
It is an integer value. It specifies the level. |
errors |
It is a string. It specifies the errors. It accepts two options: raise or ignore . If its value is raise then it allows exceptions to be raised. If its value is ignore then it ignores the exceptions and returns the original object if there is an error. |
try_cast |
It is a boolean value. It casts the output of the function to the original input type if possible. |
Return
It returns the changed DataFrame
depending upon the condition.
Example Codes: DataFrame.where()
We will learn more about this function by implementing it in the next code example.
import pandas as pd
dataframe=pd.DataFrame({
'A':
{0: 60,
1: 100,
2: 80,
3: 78,
4: 95,
5: 45,
6: 67,
7: 12,
8: 23,
9: 50},
'B':
{0: 90,
1: 75,
2: 82,
3: 64,
4: 45,
5: 35,
6: 74,
7: 52,
8: 93,
9: 18}
})
print(dataframe)
The example DataFrame
is,
A B
0 60 90
1 100 75
2 80 82
3 78 64
4 95 45
5 45 35
6 67 74
7 12 52
8 23 93
9 50 18
This function has one mandatory parameter i.e cond
.
import pandas as pd
dataframe = pd.DataFrame(
{
"A": {0: 60, 1: 100, 2: 80, 3: 78, 4: 95, 5: 45, 6: 67, 7: 12, 8: 23, 9: 50},
"B": {0: 90, 1: 75, 2: 82, 3: 64, 4: 45, 5: 35, 6: 74, 7: 52, 8: 93, 9: 18},
}
)
dataframe1 = dataframe.where(dataframe > 50)
print(dataframe1)
Output:
A B
0 60.0 90.0
1 100.0 75.0
2 80.0 82.0
3 78.0 64.0
4 95.0 NaN
5 NaN NaN
6 67.0 74.0
7 NaN 52.0
8 NaN 93.0
9 NaN NaN
The values that are not greater than 50 i.e that are not satisfying the condition are replaced by a NaN
value.
Example Codes: DataFrame.where()
to Specify a Value
import pandas as pd
dataframe = pd.DataFrame(
{
"A": {0: 60, 1: 100, 2: 80, 3: 78, 4: 95, 5: 45, 6: 67, 7: 12, 8: 23, 9: 50},
"B": {0: 90, 1: 75, 2: 82, 3: 64, 4: 45, 5: 35, 6: 74, 7: 52, 8: 93, 9: 18},
}
)
dataframe1 = dataframe.where(dataframe > 50, other=0)
print(dataframe1)
Output:
A B
0 60 90
1 100 75
2 80 82
3 78 64
4 95 0
5 0 0
6 67 74
7 0 52
8 0 93
9 0 0
Here, the values that do not meet the condition are replaced by a user-defined value.
Example Codes: DataFrame.where()
to Use Multiple Conditions
import pandas as pd
dataframe = pd.DataFrame(
{
"A": {0: 60, 1: 100, 2: 80, 3: 78, 4: 95, 5: 45, 6: 67, 7: 12, 8: 23, 9: 50},
"B": {0: 90, 1: 75, 2: 82, 3: 64, 4: 45, 5: 35, 6: 74, 7: 52, 8: 93, 9: 18},
}
)
dataframe1 = dataframe.where((dataframe == 80) | (dataframe < 50), other=0)
print(dataframe1)
Output:
A B
0 0 0
1 0 0
2 80 0
3 0 0
4 0 45
5 45 35
6 0 0
7 12 0
8 23 0
9 0 18
The returned DataFrame
contains the values that meet both of the conditions.