Pandas DataFrame DataFrame.reindex() Function
-
Syntax of
pandas.DataFrame.reindex()
-
Example Codes:
DataFrame.reindex()
-
Example Codes:
DataFrame.reindex()
to Reindex the Columns -
Example Codes:
DataFrame.reindex()
Method to Fill the Missing Values Withfill_value
-
Example Codes:
DataFrame.reindex()
to Fill the Missing Values Usingmethod
Parameter
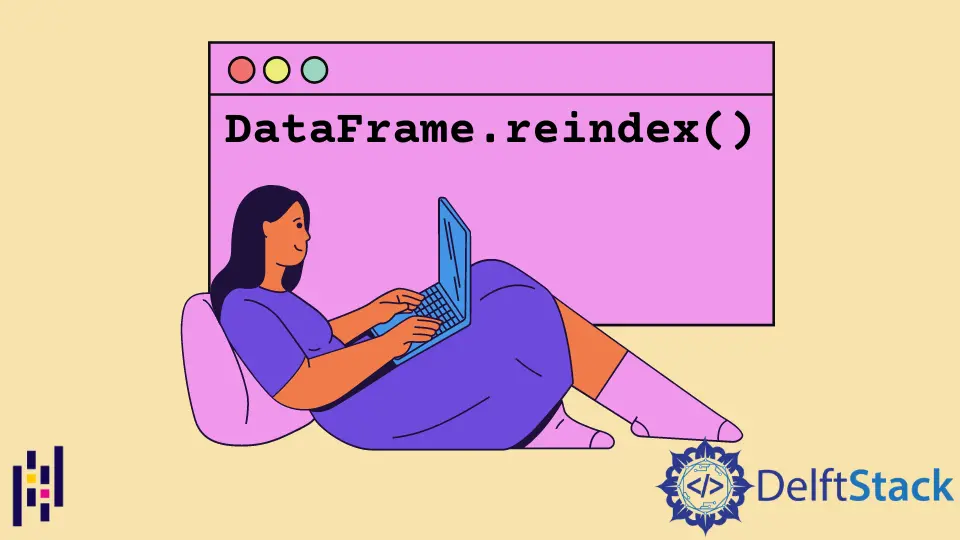
Python Pandas DataFrame.reindex()
function changes the index of a DataFrame
. It changes the indexes over the specified axis. The new indexes do not contain values. It provides optional parameters to fill in these values.
Syntax of pandas.DataFrame.reindex()
DataFrame.dropna(
labels, index, columns, axis, method, copy, level, fill_value, limit, tolerance
)
Parameters
labels |
It is an array-like structure that contains the names of the new indexes. |
index, columns |
It is an array-like structure that contains the names of the new indexes. It should be specified using the keyword index or columns . |
axis |
It is an integer or a string. It specifies the target axis either rows or columns. It can be 0 or 'index' and 1 or 'columns' . |
method |
This parameter specifies the method to fill the missing values in the reindexed DataFrame . It has four possibilities: None , backfill /bfill , pad /ffill , nearest . It only applies if our DataFrame or Series has a sequence of an increasing or decreasing index. |
copy |
Boolean . By default, it is True . It returns a new object. |
level |
It is an integer or a name. It matches index values on a passed multi-index level. |
fill_value |
It has a scalar value. It is the value to fill the missing values. |
limit |
It is an integer. It specifies the limit of the consecutive elements while filling the missing values. |
tolerance |
It specifies the difference between the original and the new labels in case of inexact matches. |
Return
It returns a DataFrame
with the changed indexes.
Example Codes: DataFrame.reindex()
By default, the axis is 0
i.e rows, so the rows will be reindexed.
import pandas as pd
dataframe=pd.DataFrame({'Attendance': {0: 60, 1: 100, 2: 80,3: 75, 4: 95},
'Name': {0: 'Olivia', 1: 'John', 2: 'Laura',3: 'Ben',4: 'Kevin'},
'Obtained Marks': {0: 56, 1: 75, 2: 82, 3: 64, 4: 67}})
print(dataframe)
The demo dataframe is as follows.
Attendance Name Obtained Marks
0 60 Olivia 56
1 100 John 75
2 80 Laura 82
3 75 Ben 64
4 95 Kevin 67
The indexes in Python starts from 0. We will reindex our DataFrame
and the new indexes will start from 1.
import pandas as pd
dataframe = pd.DataFrame(
{
"Attendance": {0: 60, 1: 100, 2: 80, 3: 75, 4: 95},
"Name": {0: "Olivia", 1: "John", 2: "Laura", 3: "Ben", 4: "Kevin"},
"Obtained Marks": {0: 56, 1: 75, 2: 82, 3: 64, 4: 67},
}
)
dataframe1 = dataframe.reindex([1, 2, 3, 4, 5])
print(dataframe1)
Output:
Attendance Name Obtained Marks
1 100.0 John 75.0
2 80.0 Laura 82.0
3 75.0 Ben 64.0
4 95.0 Kevin 67.0
5 NaN NaN NaN
Here, 5 is a new index. So, the values of the new index are NaN
.
Example Codes: DataFrame.reindex()
to Reindex the Columns
There are two ways of reindexing the columns. One is by specifying labels with the columns
keyword and the other is by using the axis parameter. The better one is to specify the labels with the columns
keyword.
import pandas as pd
dataframe = pd.DataFrame(
{
"Attendance": {0: 60, 1: 100, 2: 80, 3: 75, 4: 95},
"Name": {0: "Olivia", 1: "John", 2: "Laura", 3: "Ben", 4: "Kevin"},
"Obtained Marks": {0: 56, 1: 75, 2: 82, 3: 64, 4: 67},
}
)
dataframe1 = dataframe.reindex(columns=["Presents", "Name", "Marks"])
print(dataframe1)
Output:
Presents Name Marks
0 NaN Olivia NaN
1 NaN John NaN
2 NaN Laura NaN
3 NaN Ben NaN
4 NaN Kevin NaN
The old index is assigned with the old values. The new indexes have NaN
values.
We could also reindex the columns with the axis
parameter.
import pandas as pd
dataframe = pd.DataFrame(
{
"Attendance": {0: 60, 1: 100, 2: 80, 3: 75, 4: 95},
"Name": {0: "Olivia", 1: "John", 2: "Laura", 3: "Ben", 4: "Kevin"},
"Obtained Marks": {0: 56, 1: 75, 2: 82, 3: 64, 4: 67},
}
)
dataframe1 = dataframe.reindex(["Presents", "Name", "Marks"], axis="columns")
print(dataframe1)
Example Codes: DataFrame.reindex()
Method to Fill the Missing Values With fill_value
import pandas as pd
dataframe = pd.DataFrame(
{
"Attendance": {0: 60, 1: 100, 2: 80, 3: 75, 4: 95},
"Name": {0: "Olivia", 1: "John", 2: "Laura", 3: "Ben", 4: "Kevin"},
"Obtained Marks": {0: 56, 1: 75, 2: 82, 3: 64, 4: 67},
}
)
dataframe1 = dataframe.reindex([1, 2, 3, 4, 5], fill_value=0)
print(dataframe1)
Output:
Attendance Name Obtained Marks
1 100 John 75
2 80 Laura 82
3 75 Ben 64
4 95 Kevin 67
5 0 0 0
The missing values are now filled with 0.
Example Codes: DataFrame.reindex()
to Fill the Missing Values Using method
Parameter
import pandas as pd
dataframe = pd.DataFrame(
{
"Attendance": {0: 60, 1: 100, 2: 80, 3: 75, 4: 95},
"Name": {0: "Olivia", 1: "John", 2: "Laura", 3: "Ben", 4: "Kevin"},
"Obtained Marks": {0: 56, 1: 75, 2: 82, 3: 64, 4: 67},
}
)
dataframe1 = dataframe.reindex([1, 2, 3, 4, 5], method="ffill")
print(dataframe1)
Output:
Attendance Name Obtained Marks
1 100 John 75
2 80 Laura 82
3 75 Ben 64
4 95 Kevin 67
5 95 Kevin 67
The method ffill
has filled the forward missing values with the last available value.