Pandas DataFrame DataFrame.min() Function
-
Syntax of
pandas.DataFrame.min()
: -
Example Codes:
DataFrame.min()
Method to Find Min Along Column Axis -
Example Codes:
DataFrame.min()
Method to Find Min Along Row Axis -
Example Codes:
DataFrame.min()
Method to Find Min IgnoringNaN
Values
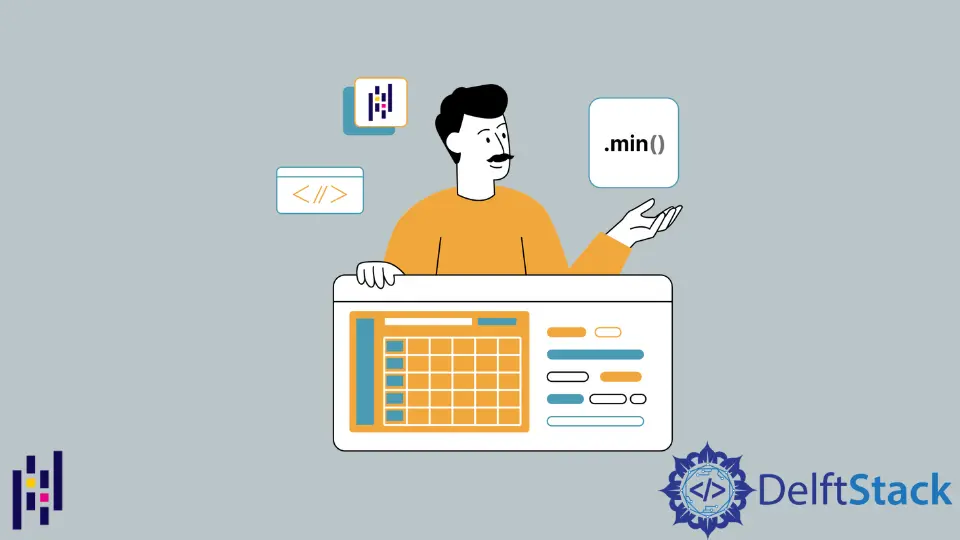
Python Pandas DataFrame.min()
function gets the minimum of values of DataFrame object over the specified axis.
Syntax of pandas.DataFrame.min()
:
DataFrame.mean(axis=None, skipna=None, level=None, numeric_only=None, **kwargs)
Parameters
axis |
find mean along the row (axis=0) or column (axis=1) |
skipna |
Boolean. Exclude NaN values (skipna=True ) or include NaN values (skipna=False ) |
level |
Count along with particular level if the axis is MultiIndex |
numeric_only |
Boolean. For numeric_only=True , include only float , int , and boolean columns |
**kwargs |
Additional keyword arguments to the function. |
Return
If the level
is not specified, return Series
of the minimum of the values for the requested axis, else return DataFrame
of minimum values.
Example Codes: DataFrame.min()
Method to Find Min Along Column Axis
import pandas as pd
df = pd.DataFrame({'X': [1, 2, 2, 3],
'Y': [4, 3, 8, 4]})
print("DataFrame:")
print(df)
mins = df.min()
print("Min of Each Column:")
print(mins)
Output:
DataFrame:
X Y
0 1 4
1 2 3
2 2 8
3 3 4
Min of Each Column:
X 1
Y 3
dtype: int64
It gets the min value for both columns X
and Y
and finally returns a Series
object with the min of each column.
To find the min of a particular column of DataFrame
in Pandas, we call the min()
function for that column only.
import pandas as pd
df = pd.DataFrame({'X': [1, 2, 2, 3],
'Y': [4, 3, 8, 4]})
print("DataFrame:")
print(df)
mins = df["X"].min()
print("Min of Each Column:")
print(mins)
Output:
1DataFrame:
X Y
0 1 4
1 2 3
2 2 8
3 3 4
Min of Each Column:
1
It only gives the min of values of column X
in the DataFrame
.
Example Codes: DataFrame.min()
Method to Find Min Along Row Axis
import pandas as pd
df = pd.DataFrame({'X': [1, 2, 7, 5, 10],
'Y': [4, 3, 8, 2, 9],
'Z': [2, 7, 6, 10, 5]})
print("DataFrame:")
print(df)
mins=df.min(axis=1)
print("Min of Each Row:")
print(mins)
Output:
DataFrame:
X Y Z
0 1 4 2
1 2 3 7
2 7 8 6
3 5 2 10
4 10 9 5
Min of Each Row:
0 1
1 2
2 6
3 2
4 5
dtype: int64
It calculates the min for all the rows and finally returns a Series
object with the mean of each row.
Example Codes: DataFrame.min()
Method to Find Min Ignoring NaN
Values
We use the default value of skipna
parameter i.e. skipna=True
to find the min of DataFrame
along the specified axis ignoring NaN
values.
import pandas as pd
df = pd.DataFrame({'X': [1, 2, None, 3],
'Y': [4, 3, 7, 4]})
print("DataFrame:")
print(df)
mins=df.min(skipna=True)
print("Min of Columns")
print(mins)
Output:
DataFrame:
X Y
0 1.0 4.0
1 2.0 3.0
2 NaN 7.0
3 3.0 4.0
Min of Columns
X 1.0
Y 3.0
dtype: float64
If we set skipna=True
, it ignores the NaN
in the dataframe. It allows us to calculate the min of DataFrame
along column axis ignoring NaN
values.
import pandas as pd
df = pd.DataFrame({'X': [1, 2, None, 3],
'Y': [4, 3, 7, 4]})
print("DataFrame:")
print(df)
mins=df.min(skipna=False)
print("Min of Columns")
print(mins)
Output:
DataFrame:
X Y
0 1.0 4
1 2.0 3
2 NaN 7
3 3.0 4
Min of Columns
X NaN
Y 3.0
dtype: float64
Here, we get NaN
value for the mean of column X
as column X
has NaN
value present in it.
Founder of DelftStack.com. Jinku has worked in the robotics and automotive industries for over 8 years. He sharpened his coding skills when he needed to do the automatic testing, data collection from remote servers and report creation from the endurance test. He is from an electrical/electronics engineering background but has expanded his interest to embedded electronics, embedded programming and front-/back-end programming.
LinkedIn Facebook